1. Overview
The REST API enables you to develop, integrate, and deploy secure file transfer and management applications that leverage your organization or system-wide MOVEit Transfer solution.
Using the Transfer REST API (instead of the Transfer .NET or Java API) enables you to connect systems and clients to MOVEit Transfer using simple HTTP calls. In general, REST APIs are language and platform independent and can be your best choice to converge information systems, circumvent the unending need for client-server dependency maintenance, and span any combination of environments (including IoT, mobile, and much more).
Tip
|
The REST API runs at the MOVEit Transfer Server (for example: https://<your-transfer-server>/api/v1/). |
Note
|
In general, this RESTful API expects the following verbs: GET (query record), POST (create/delete record), PATCH (modify/update record), or DELETE (remove record). |
This REST Endpoint | Provides These Resources | |
---|---|---|
1. |
Get/renew a session token using MOVEit Transfer user credentials. |
|
2. |
Create and manage users and groups, roles, home folders, and other properties. |
|
3. |
Folders, folder actions, folder contents and folder properties. |
|
4. |
File management actions and file properties. |
|
5. |
Get status and summaries for ongoing and recently completed transfers. |
|
6. |
Get information about a MOVEit organization. |
|
7. |
Live Swagger UI interactive documentation (MOVEit Transfer installation required). |
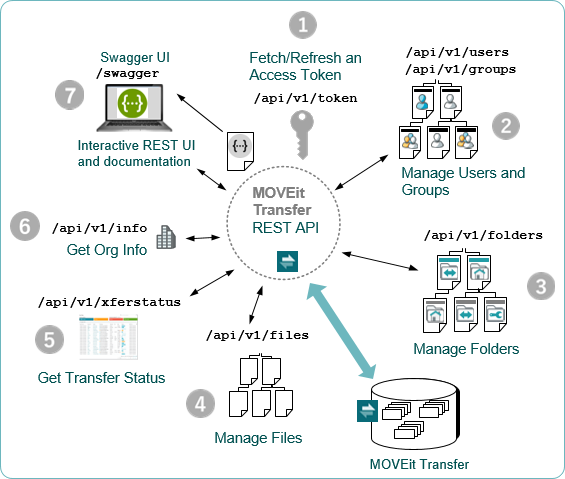
2. Before You Begin
Before you begin using the MOVEit Transfer RESTful API, you will need the following:
-
The hostname for a deployed MOVEit Transfer 2019 system.
-
Username and password for a user on the target MOVEit Transfer system.
-
Your favorite HTTP client (the examples that follow use the cURL utility).
Listener | URL | Purpose |
---|---|---|
Swagger UI |
Browsable/interactive UI service available for exercising the REST API. |
|
REST API |
Primary REST API Endpoint. |
2.1. A quick check of the API
If you are unsure whether or not the REST API is already listening for requests on a MOVEit Transfer host —here is a quick check. Type into your web browser https://<your-transfer-server>/api/v1/token
. (Where <your-transfer-server>
is the hostname of the MOVEit Transfer system.) If this call returns a simple JSON object, your API is running, and you can begin the steps that follow using this hostname.
Tip
|
You can also use the Swagger spec object (https://<your-transfer-server>/api/v1/swagger ) in tools such as Postman, Swagger Inspector, and more.
|
2.2. Interactive REST API Client - Swagger UI
Swagger UI libraries integrated with MOVEit Transfer at install time provide the following:
-
A way to browse the MOVEit Transfer REST documentation with live examples.
-
A simple development client for testing MOVEit Transfer REST calls.
Caution
|
The examples are live calls (not simulated) that run against your database. If you are just testing and exploring the capabilities of the REST API for development purposes, it is best practice to run against a non-production instance of MOVEit Transfer. |
To open the interactive MOVEit Transfer REST client:
-
Open a browser to
https://my-transfer-host/swagger/
--Wheremy-transfer-host
is the host where you installed MOVEit Transfer -
Browse and build queries and view JSON result objects.
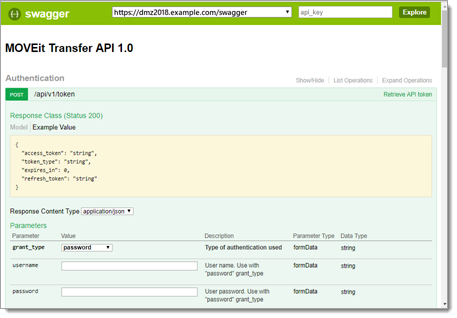
3. Getting Started
This section walks you through how to fetch user properties for the current user.
Tip
|
If you are running an API token request against a non-production host with a self-signed SSL certificate needed to run HTTPS, remember to set the flag to ignore the self-signed certificate warning. (In the cURL example shown below this is the -k option.)
|
Step 1: Request access token
To set your session up with the MOVEit Transfer RESTful API, you must retrieve an access token. (Syntax, usage, and fields supported by the token endpoint are detailed in Retrieve API Token and Revoke API token.)
curl -k --request POST --url https://your-transfer-server/api/v1/token --data "grant_type=password&username=emmacurtis&password=1a2B3cA1b2C3"
Example Output
{
"access_token": "X03w.....dziTwmA",
"token_type": "bearer",
"expires_in": 86399,
"refresh_token": "X03w.....dziTwmA"
}
--Where your-transfer-server
is the hostname where your MOVEit Transfer WebUI is running.
--And, where you supply values for the username and password (such as the MOVEit user you created for this RESTful client application).
Step 2: Get my user ID
Now, pass the access token as a header argument, type "Bearer" and get your user information.
curl -H "Authorization: Bearer X03w.....dziTwmA" "https://your-transfer-server/api/v1/users/self"
--Where your-transfer-server
is the hostname where your MOVEit Transfer WebUI is running.
--And, where you should replace the placeholder string X03w…..dziTwmA
with your 278 Byte access token.
Example Output
{
"emailFormat": "HTML",
"notes": "",
"statusNote": "",
"passwordChangeStamp": "2017-04-17T09:36:47",
"receivesNotification": "ReceivesNotifications",
"forceChangePassword": false,
"folderQuota": 0,
"authMethod": "MOVEitOnly",
"language": "en",
"homeFolderID": 377392666,
"defaultFolderID": 377392666,
"expirationPolicyID": 0,
"displaySettings": {
"userListPageSize": 10,
"fileListPageSize": 100,
"liveViewPageSize": 25,
"id": "axaxfbz5v85l7dp1",
"orgID": 9683,
"username": "org1admin",
"realname": "Denise Taylor",
"permission": "Admin",
"email": "dtaylor@example.com",
"status": "Active",
"lastLoginStamp": "2018-04-06T16:13:50"
}
Step 3: List files in my home folder
Now, use the homeFolderID value (311392888
) and get a list of files in the folder.
curl -H "Authorization: Bearer your-access-token" "https://your-transfer-server/api/v1/folders/311392888/files"
Example Output
{
"items": [
{
"id": "455123456",
"name": "iMacros-for-Chrome-File-Access-Setup-Mac.dmg",
"path": "/Home/org1admin/iMacros-for-Chrome-File-Access-Setup-Mac.dmg",
"fileSize": 4454619,
"uploadStamp": "2018-02-08T14:48:08"
},
{
"id": "455123455",
"name": "MOVEit-Client-0.1.0-mac.zip",
"path": "/Home/org1admin/MOVEit-Client-0.1.0-mac.zip",
"fileSize": 61725986,
"uploadStamp": "2018-02-08T08:58:45"
}
],
"currentPage": 1,
"totalItems": 2,
"itemsPerPage": 25,
"sorting": [
{
"sortField": "name",
"sortDirection": "asc"
}
]
}
4. Handling Session Tokens
Before you can be authorized to access MOVEit Transfer resources using this RESTful API, you must request an access token. Only authenticated clients can fetch or renew an access token.
4.1. Request/refresh an access token
HTTPS sessions with MOVEit Transfer begin with a token request. It must be sent as an HTTP POST.
POST /api/v{version}/token
4.1.1. Request Example
Tip
|
If you are running an API token request against a non-production host with a self-signed SSL certificate needed to run HTTPS, remember to set the flag to ignore the self-signed certificate warning. (In the cURL example shown below this is the -k option.)
|
curl -k --request POST --url https://mydmzserver/api/v1/token --data "grant_type=password&username=${username}&password=${password}"
--Where mydmzserver
is the hostname where your MOVEit Transfer WebUI is running.
--And, where ${username}
and ${password}
are the username and password you created for the current client application.
Note
|
For logging purposes, it is best practice to create an new API user using the MOVEit Transfer WebUI. |
4.1.2. Refresh Example
curl -X POST https://mydmzserver/api/v1/token -H "accept: application/json" -H "Content-Type: application/x-www-form-urlencoded" -d "grant_type=refresh_token&refresh_token=${refresh-token}"
--Where mydmzserver
is the hostname where your MOVEit Transfer WebUI is running.
--And, where ${refresh-token}
is the token received from the last authenticated token request (this request must be submitted within the allowed expiration period).
Tip
|
Token expiration duration is determined by client session properties configured at the MOVEit Transfer Server. |
4.2. Token response messages
Response Content Type: application/json
HTTP Status Code | Meaning | Response Model |
---|---|---|
200 |
SUCCESS |
|
400 |
BAD REQUEST |
|
4.3. Use token in request
After you get your session token you can use the token in subsequent requests. You pass the token in the HTTP header as type Bearer
. For example, to get information
such as user directory, name, user ID, and more for the current user, you would send this cURL GET request that passes the token using the -H
option.
4.3.1. Example
curl -H "Authorization: Bearer X03w....dziTwmA" 'https://mydmzserver/api/v1/users/self"
--Where mydmzserver
is the hostname where your MOVEit Transfer WebUI is running.
5. Paths
5.1. Get list of files current user can view
GET /api/v1/files
5.1.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Query |
page |
Page number to display |
integer (int32) |
Query |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
Query |
sortDirection |
Sort direction; Default is ascending. |
string |
Query |
sortField |
Name of field to sort the results by. (name, size, datetime, uploadstamp or path); Default is Path. |
string |
5.1.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.1.3. Produces
-
application/json
-
text/json
5.1.4. Tags
-
Files
5.1.5. Security
Type | Name |
---|---|
apiKey |
5.2. Return file details
GET /api/v1/files/{Id}
5.2.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
File ID |
string |
5.2.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.2.3. Produces
-
application/json
-
text/json
5.2.4. Tags
-
Files
5.2.5. Security
Type | Name |
---|---|
apiKey |
5.3. Delete file
DELETE /api/v1/files/{Id}
5.3.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
File ID |
string |
5.3.2. Responses
HTTP Code | Description | Schema |
---|---|---|
204 |
No Content |
|
403 |
Forbidden |
|
500 |
Server Error |
5.3.3. Produces
-
application/json
-
text/json
5.3.4. Tags
-
Files
5.3.5. Security
Type | Name |
---|---|
apiKey |
5.4. Partial file update
PATCH /api/v1/files/{Id}
5.4.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
ID of the file to update |
string |
Body |
body |
Request body. |
5.4.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.4.3. Consumes
-
application/json
-
text/json
5.4.4. Produces
-
application/json
-
text/json
5.4.5. Tags
-
Files
5.4.6. Security
Type | Name |
---|---|
apiKey |
5.5. Copy file into another folder
POST /api/v1/files/{Id}/copy
5.5.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
ID of the file to copy |
string |
Body |
body |
File copy request details |
5.5.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
404 |
Resource Not Found |
|
409 |
Operation Conflicted |
|
500 |
Server Error |
5.5.3. Consumes
-
application/json
-
text/json
5.5.4. Produces
-
application/json
-
text/json
5.5.5. Tags
-
Files
5.5.6. Security
Type | Name |
---|---|
apiKey |
5.6. Download file
GET /api/v1/files/{Id}/download
5.6.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
File ID |
string |
5.6.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
file |
403 |
Forbidden |
|
500 |
Server Error |
5.6.3. Produces
-
application/octet-stream
5.6.4. Tags
-
Files
5.6.5. Security
Type | Name |
---|---|
apiKey |
5.7. Get list of folders current user can view
GET /api/v1/folders
5.7.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Query |
name |
File name mask for filtering list of Folders |
string |
Query |
page |
Page number to display |
integer (int32) |
Query |
path |
File path mask for filtering list of Folders |
string |
Query |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
Query |
sortDirection |
Sort direction; Default is ascending. |
string |
Query |
sortField |
Name of field to sort the results by. (Name, Type or Path); Default is Path. |
string |
5.7.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.7.3. Produces
-
application/json
-
text/json
5.7.4. Tags
-
Folders
5.7.5. Security
Type | Name |
---|---|
apiKey |
5.8. Return folder details
GET /api/v1/folders/{Id}
5.8.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
Folder ID |
string |
5.8.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.8.3. Produces
-
application/json
-
text/json
5.8.4. Tags
-
Folders
5.8.5. Security
Type | Name |
---|---|
apiKey |
5.9. Delete folder
DELETE /api/v1/folders/{Id}
5.9.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
Folder ID |
string |
5.9.2. Responses
HTTP Code | Description | Schema |
---|---|---|
204 |
No Content |
|
403 |
Forbidden |
|
500 |
Server Error |
5.9.3. Produces
-
application/json
-
text/json
5.9.4. Tags
-
Folders
5.9.5. Security
Type | Name |
---|---|
apiKey |
5.10. Partial Folder update
PATCH /api/v1/folders/{Id}
5.10.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
ID of the folder to update |
string |
Body |
body |
Request body. |
5.10.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.10.3. Consumes
-
application/json
-
text/json
5.10.4. Produces
-
application/json
-
text/json
5.10.5. Tags
-
Folders
5.10.6. Security
Type | Name |
---|---|
apiKey |
5.11. Set the Access Controls for a given folder
POST /api/v1/folders/{Id}/acls
5.11.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
ID of the folder to set access control |
string |
Body |
body |
Access control |
5.11.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.11.3. Consumes
-
application/json
-
text/json
5.11.4. Produces
-
application/json
-
text/json
5.11.5. Tags
-
Folders
5.11.6. Security
Type | Name |
---|---|
apiKey |
5.12. Lists the Access Controls for a given folder
GET /api/v1/folders/{Id}/acls
5.12.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
Folder ID |
string |
Query |
page |
Page number to display |
integer (int32) |
Query |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
Query |
sortDirection |
Sort direction; Default is ascending. |
string |
Query |
sortField |
Field to sort by; Default is name. |
string |
5.12.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.12.3. Produces
-
application/json
-
text/json
5.12.4. Tags
-
Folders
5.12.5. Security
Type | Name |
---|---|
apiKey |
5.13. Delete the Access Controls for a given folder
DELETE /api/v1/folders/{Id}/acls
5.13.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
ID of the folder to delete access control |
string |
Body |
body |
Entity type and id |
5.13.2. Responses
HTTP Code | Description | Schema |
---|---|---|
204 |
No Content |
|
403 |
Forbidden |
|
500 |
Server Error |
5.13.3. Consumes
-
application/json
-
text/json
5.13.4. Produces
-
application/json
-
text/json
5.13.5. Tags
-
Folders
5.13.6. Security
Type | Name |
---|---|
apiKey |
5.14. Get content of the folder
GET /api/v1/folders/{Id}/content
5.14.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
Parent Folder ID |
string |
Query |
name |
Name mask to search by |
string |
Query |
page |
Page number to display |
integer (int32) |
Query |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
Query |
sortDirection |
Sort direction; Default is ascending. |
string |
Query |
sortField |
Field to sort by; (type, time, size or name); Sorting order starts with type and ends with name |
string |
5.14.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.14.3. Produces
-
application/json
-
text/json
5.14.4. Tags
-
FolderContent
5.14.5. Security
Type | Name |
---|---|
apiKey |
5.15. Upload file into folder
POST /api/v1/folders/{Id}/files
5.15.1. Description
The content type of the request must be "multipart/form-data". For large files upload (larger then maxAllowedContentLength attribute in Web.config) use chunked upload. Some clients, like Swagger and Postman, are not using chunked upload, so the upload might fail with unexpected error (404.13) More info how to setup chunked upload: https://www.ipswitch.com/Transfer2018/ChunkedUploaderExample/
5.15.2. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
Folder ID |
string |
FormData |
comments |
File upload comments |
string |
FormData |
file |
File contents |
file |
FormData |
hash |
Hash of file |
string |
FormData |
hashtype |
Type of hash provided (sha-1, sha-256, sha-384, sha-512) |
string |
5.15.3. Responses
HTTP Code | Description | Schema |
---|---|---|
201 |
Created |
|
403 |
Forbidden |
|
422 |
Invalid Request Parameters |
|
500 |
Server Error |
5.15.4. Consumes
-
multipart/form-data
5.15.5. Produces
-
application/json
-
text/json
5.15.6. Tags
-
FolderContent
5.15.7. Security
Type | Name |
---|---|
apiKey |
5.16. Get list of files in folder
GET /api/v1/folders/{Id}/files
5.16.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
Parent Folder ID |
string |
Query |
name |
File Name mask to search by |
string |
Query |
page |
Page number to display |
integer (int32) |
Query |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
Query |
sortDirection |
Sort direction; Default is ascending. |
string |
Query |
sortField |
Field to sort by; Default is name. |
string |
5.16.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.16.3. Produces
-
application/json
-
text/json
5.16.4. Tags
-
FolderContent
5.16.5. Security
Type | Name |
---|---|
apiKey |
5.17. Create new subfolder in folder
POST /api/v1/folders/{Id}/subfolders
5.17.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
ID of the folder where new folder should be added |
string |
Body |
body |
Request body. |
5.17.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.17.3. Consumes
-
application/json
-
text/json
5.17.4. Produces
-
application/json
-
text/json
5.17.5. Tags
-
FolderContent
5.17.6. Security
Type | Name |
---|---|
apiKey |
5.18. Get list of subfolders in folder
GET /api/v1/folders/{Id}/subfolders
5.18.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
Parent Folder ID |
string |
Query |
name |
Folder Name mask to search by |
string |
Query |
page |
Page number to display |
integer (int32) |
Query |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
Query |
sortDirection |
Sort direction; Default is ascending. |
string |
Query |
sortField |
Field to sort by; Default is name. |
string |
5.18.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.18.3. Produces
-
application/json
-
text/json
5.18.4. Tags
-
FolderContent
5.18.5. Security
Type | Name |
---|---|
apiKey |
5.19. Get file details in folder. Redirect
GET /api/v1/folders/{folderId}/files/{fileId}
5.19.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
fileId |
File ID |
string |
Path |
folderId |
Parent folder ID |
string |
5.19.2. Responses
HTTP Code | Description | Schema |
---|---|---|
302 |
Redirect |
No Content |
403 |
Forbidden |
|
500 |
Server Error |
5.19.3. Produces
-
application/json
-
text/json
5.19.4. Tags
-
FolderContent
5.19.5. Security
Type | Name |
---|---|
apiKey |
5.20. Download file. Redirect
GET /api/v1/folders/{folderId}/files/{fileId}/download
5.20.1. Description
The response header includes information about base64-encoded SHA1 digest value returned with file download response
5.20.2. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
fileId |
File ID |
string |
Path |
folderId |
Parent folder ID |
string |
5.20.3. Responses
HTTP Code | Description | Schema |
---|---|---|
302 |
Redirect |
No Content |
403 |
Forbidden |
|
422 |
Invalid Request Parameters |
|
500 |
Server Error |
5.20.4. Produces
-
application/json
-
text/json
5.20.5. Tags
-
FolderContent
5.20.6. Security
Type | Name |
---|---|
apiKey |
5.21. Get subfolder details. Redirect
GET /api/v1/folders/{parentId}/subfolders/{subfolderId}
5.21.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
parentId |
Parent folder ID |
string |
Path |
subfolderId |
Folder ID to Get |
string |
5.21.2. Responses
HTTP Code | Description | Schema |
---|---|---|
302 |
Redirect |
No Content |
403 |
Forbidden |
|
500 |
Server Error |
5.21.3. Produces
-
application/json
-
text/json
5.21.4. Tags
-
FolderContent
5.21.5. Security
Type | Name |
---|---|
apiKey |
5.22. Delete subfolder. Redirect
DELETE /api/v1/folders/{parentId}/subfolders/{subfolderId}
5.22.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
parentId |
Parent folder ID |
string |
Path |
subfolderId |
Folder ID to Delete |
string |
5.22.2. Responses
HTTP Code | Description | Schema |
---|---|---|
302 |
Redirect |
No Content |
403 |
Forbidden |
|
500 |
Server Error |
5.22.3. Produces
-
application/json
-
text/json
5.22.4. Tags
-
FolderContent
5.22.5. Security
Type | Name |
---|---|
apiKey |
5.23. Create new group
POST /api/v1/groups
5.23.1. Parameters
Type | Name | Schema |
---|---|---|
Body |
body |
5.23.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
5.23.3. Consumes
-
application/json
-
text/json
5.23.4. Produces
-
application/json
-
text/json
5.23.5. Tags
-
Groups
5.23.6. Security
Type | Name |
---|---|
apiKey |
5.24. Get list of groups current user can view
GET /api/v1/groups
5.24.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Query |
page |
Page number to display |
integer (int32) |
Query |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
5.24.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
5.24.3. Produces
-
application/json
-
text/json
5.24.4. Tags
-
Groups
5.24.5. Security
Type | Name |
---|---|
apiKey |
5.25. Get a list of the members of a group
GET /api/v1/groups/{Id}/members
5.25.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
Group ID |
string |
Query |
page |
Page number to display |
integer (int32) |
Query |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
5.25.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
5.25.3. Produces
-
application/json
-
text/json
5.25.4. Tags
-
Groups
5.25.5. Security
Type | Name |
---|---|
apiKey |
5.26. Gets information about organization
GET /api/v1/info
5.26.1. Parameters
Type | Name | Description | Schema | Default |
---|---|---|---|---|
Query |
orgId |
Organization ID |
string |
|
5.26.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
500 |
Server Error |
5.26.3. Produces
-
application/json
-
text/json
5.26.4. Tags
-
OrganizationInfo
5.26.5. Security
Type | Name |
---|---|
apiKey |
5.27. Retrieve API token
POST /api/v1/token
5.27.1. Description
Retrieve API token
5.27.2. Parameters
Type | Name | Description | Schema |
---|---|---|---|
FormData |
grant_type |
Type of authentication used |
enum (password, refresh_token) |
FormData |
language |
Language to use. Optional. Use with "password" grant_type |
string |
FormData |
orgId |
Organization ID to authenticate against. Optional. Use with "password" grant_type |
string |
FormData |
password |
User password. Use with "password" grant_type |
string |
FormData |
refresh_token |
Refresh token. Use with "refresh_token" grant_type |
string |
FormData |
username |
User name. Use with "password" grant_type |
string |
5.27.3. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
Represents successful token request response |
|
400 |
Authentication failed error |
5.27.4. Consumes
-
application/x-www-form-urlencoded
5.27.5. Tags
-
Authentication
5.28. Revokes API token
POST /api/v1/token/revoke
5.28.1. Description
Response for Revoke token operation
5.28.2. Parameters
Type | Name | Description | Schema |
---|---|---|---|
FormData |
token |
Access token or Refresh token to revoke |
string |
5.28.3. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
401 |
Unauthorized |
5.28.4. Consumes
-
application/x-www-form-urlencoded
5.28.5. Tags
-
Authentication
5.29. Create new user
POST /api/v1/users
5.29.1. Description
If SourceUserId specified in user model clone operation will be executed
5.29.2. Parameters
Type | Name | Schema |
---|---|---|
Body |
body |
5.29.3. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.29.4. Consumes
-
application/json
-
text/json
5.29.5. Produces
-
application/json
-
text/json
5.29.6. Tags
-
Users
5.29.7. Security
Type | Name |
---|---|
apiKey |
5.30. Get list of users
GET /api/v1/users
5.30.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Query |
email |
User email |
string |
Query |
fullName |
User real name |
string |
Query |
page |
Page number to display |
integer (int32) |
Query |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
Query |
permission |
User permission filter. |
enum (AllUsers, EndUsers, Administrators, FileAdmins, GroupAdmins, TemporaryUsers, SysAdmins) |
Query |
realname |
User real name |
string |
Query |
sortDirection |
Sort direction; Default is ascending. |
string |
Query |
sortField |
Name of field to sort the results by. (username, realname, lastLoginStamp or email); Default is username. |
string |
Query |
status |
User status filter. |
enum (AllUsers, ActiveUsers, InactiveUsers, NeverSignedOnUsers, TemplateUsers) |
Query |
username |
User name |
string |
5.30.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.30.3. Produces
-
application/json
-
text/json
5.30.4. Tags
-
Users
5.30.5. Security
Type | Name |
---|---|
apiKey |
5.31. Get current user details
GET /api/v1/users/self
5.31.1. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.31.2. Produces
-
application/json
-
text/json
5.31.3. Tags
-
Users
5.31.4. Security
Type | Name |
---|---|
apiKey |
5.32. Get user details
GET /api/v1/users/{Id}
5.32.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
User ID |
string |
5.32.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.32.3. Produces
-
application/json
-
text/json
5.32.4. Tags
-
Users
5.32.5. Security
Type | Name |
---|---|
apiKey |
5.33. Delete user
DELETE /api/v1/users/{Id}
5.33.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
User ID |
string |
5.33.2. Responses
HTTP Code | Description | Schema |
---|---|---|
204 |
No Content |
|
403 |
Forbidden |
|
500 |
Server Error |
5.33.3. Produces
-
application/json
-
text/json
5.33.4. Tags
-
Users
5.33.5. Security
Type | Name |
---|---|
apiKey |
5.34. Partial user update
PATCH /api/v1/users/{Id}
5.34.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
ID of user to update |
string |
Body |
body |
Request body. |
5.34.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.34.3. Consumes
-
application/json
-
text/json
5.34.4. Produces
-
application/json
-
text/json
5.34.5. Tags
-
Users
5.34.6. Security
Type | Name |
---|---|
apiKey |
5.35. Get list of user groups
GET /api/v1/users/{Id}/groups
5.35.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Path |
Id |
User Id. |
string |
Query |
page |
Page number to display |
integer (int32) |
Query |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
5.35.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.35.3. Produces
-
application/json
-
text/json
5.35.4. Tags
-
Users
5.35.5. Security
Type | Name |
---|---|
apiKey |
5.36. Gets Transfer status information
GET /api/v1/xferstatus
5.36.1. Parameters
Type | Name | Description | Schema |
---|---|---|---|
Query |
fileName |
Filter expression for File name |
string |
Query |
folderName |
Filter expression for Folder name |
string |
Query |
page |
Page number to display |
integer (int32) |
Query |
perPage |
Items per page in result collection |
integer (int32) |
Query |
recentlyCompletedPeriod |
Time period in seconds to return recently completed data. Default: 0 |
integer (int32) |
Query |
search |
Filter expression for several fields. Fields are matched with OR logic. AND logic is used if you provide expression for each particular query. Fields being matched: userLoginName, userRealName, userIp, folderName, fileName |
string |
Query |
sortDirection |
Sort direction; Default is ascending. |
string |
Query |
sortField |
Name of field to sort the results by. |
string |
Query |
statusDistributionPeriod |
Time period in seconds to calculate status distribution for. Default: RecentlyCompletedPeriod |
integer (int32) |
Query |
transferStatus |
Filter for status. Uses OR logic |
< enum (Failed, Stalled, Active, Completed) > array(multi) |
Query |
userFullName |
Filter expression for User Display name |
string |
Query |
userIp |
Filter expression for User IP |
string |
Query |
userLoginName |
Filter expression for Login name |
string |
Query |
userRealName |
Filter expression for User Display name |
string |
5.36.2. Responses
HTTP Code | Description | Schema |
---|---|---|
200 |
OK |
|
403 |
Forbidden |
|
500 |
Server Error |
5.36.3. Produces
-
application/json
-
text/json
5.36.4. Tags
-
TransferStatus
5.36.5. Security
Type | Name |
---|---|
apiKey |
6. Definitions
6.1. AddFolderModel
Name | Description | Schema |
---|---|---|
inheritPermissions |
Indicates how parent folder permissions should be inherited |
enum (None, CopyOnly, Always) |
name |
Folder name |
string |
6.2. AddUserModel
Name | Description | Schema |
---|---|---|
email |
User email address. |
string |
forceChangePassword |
If set to true, user must change his password on his next login. |
boolean |
fullName |
User full name. |
string |
homeFolderInUseOption |
Describes a behavior when specified home folder is already in use |
enum (AllowIfExists, DenyIfExists) |
homeFolderPath |
Path of user home folder to create. If empty no home folder will be created. |
string |
notes |
User description. |
string |
orgID |
ID of organization new user has to be member of. Will be used only when client user is SysAmin. Otherwise it will be ignored and current user orgId will be used. |
integer (int32) |
password |
User password. |
string |
permission |
User permission. |
enum (TemporaryUser, User, FileAdmin, Admin, SysAdmin) |
realName |
User full name. |
string |
sourceUserId |
Source user ID to clone from. |
string |
username |
User login name. |
string |
6.3. BaseGroupModel
Name | Description | Schema |
---|---|---|
description |
Additional information, related to the group. |
string |
id |
Unique ID for this group. |
integer (int32) |
name |
Name of the group. |
string |
6.4. DeleteFolderAclEntryModel
Represents delete folder Access Control List entry data suitable for all scenarios.
Name | Description | Schema |
---|---|---|
id |
ID of the entity referenced by the entry. |
string |
type |
Type of the entry. |
enum (User, Group) |
6.5. DisplaySettingsModel
Information about configured display setting
Name | Description | Schema |
---|---|---|
fileListPageSize |
Files/folders entries per page |
integer (int32) |
liveViewPageSize |
Live transfer entries per page |
integer (int32) |
userListPageSize |
User/group entries per page |
integer (int32) |
6.6. ErrorModel
Represents an error returned by API
Name | Description | Schema |
---|---|---|
detail |
An explanation specific to this occurrence of the error |
string |
errorCode |
An error number, defined by the application |
integer (int32) |
title |
Short summary of the error type |
string |
6.7. FieldErrorModel
Describes error in particular parameter of the request
Name | Description | Schema |
---|---|---|
field |
Name of the field that’s invalid |
string |
message |
Explanation of the error |
string |
rejected |
Value of the field that’s invalid |
string |
6.8. FileCopyRequest
File copy request details
Name | Description | Schema |
---|---|---|
destinationFolderId |
ID of the folder where to put a copy of the file |
string |
id |
File ID |
string |
6.9. FileDetailsModel
Represents a file and all available attributes
Name | Description | Schema |
---|---|---|
currentFileType |
Describes what type of file this file is currently. |
enum (Any, Text, HTML, CSV, XML, WebPost, AuditLog, Message, MessageArchive, MessageDraft, MessageTemplate, Upload31, Upload32) |
downloadCount |
Number of times this file has been downloaded. |
integer (int32) |
folderID |
ID of the folder this file is currently in. |
string |
hash |
SHA-1 hash of the file |
string |
id |
The unique ID of this file. Also known as the tracking number. |
string |
name |
The name of this file. |
string |
orgID |
ID of the organization this file exists in. |
string |
originalFileType |
Describes what type of file this file was originally. |
enum (Any, Text, HTML, CSV, XML, WebPost, AuditLog, Message, MessageArchive, MessageDraft, MessageTemplate, Upload31, Upload32) |
originalFilename |
The original name of this file. |
string |
path |
The full path of this file. |
string |
size |
Size in bytes of this file. |
integer (int64) |
uploadAgentBrand |
Brand of the agent used to upload this file. |
string |
uploadAgentVersion |
Version of the agent used to upload this file. |
string |
uploadComment |
Any optional comments uploaded with this file. |
string |
uploadIP |
The IP Address, if any, from which this file was uploaded. |
string |
uploadIntegrity |
1 if file was uploaded with integrity checking, 0 if file was not. |
integer (int32) |
uploadStamp |
The date and time this file was uploaded. |
string |
uploadUserFullName |
The real name of the user account which uploaded this file. |
string |
uploadUserRealname |
The real name of the user account which uploaded this file. |
string |
uploadUsername |
The username of the user account which uploaded this file. |
string |
6.10. FilePatchModel
File Update parameters
Name | Description | Schema |
---|---|---|
name |
New file name to set |
string |
6.11. FileRequest
Name | Description | Schema |
---|---|---|
id |
File ID |
string |
6.12. FolderAclEntryModel
Represents folder Access Control List entry data suitable for all scenarios.
Name | Description | Schema |
---|---|---|
id |
ID of the entity referenced by the entry. |
string |
name |
Name of the entity referenced by the entry. |
string |
permissions |
Permissions set on the entry. |
|
type |
Type of the entry. |
enum (User, Group) |
6.13. FolderAclPermissionsModel
Name | Schema |
---|---|
addDeleteSubfolders |
boolean |
admin |
boolean |
deleteFiles |
boolean |
listFiles |
boolean |
listUsers |
boolean |
notify |
boolean |
readFiles |
boolean |
share |
boolean |
writeFiles |
boolean |
6.14. FolderAclsRequest
Name | Description | Schema |
---|---|---|
id |
Folder ID |
string |
page |
Page number to display |
integer (int32) |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
sortDirection |
Sort direction; Default is ascending. |
string |
sortField |
Field to sort by; Default is name. |
string |
6.15. FolderContentItemModel
Represents information about items stored in folder.
Name | Description | Schema |
---|---|---|
folderType |
Indicates what kind of folder this is. |
enum (Virtual, Normal, Archive, Webpost, GlobalMessaging, Mailbox, Root, RootArchives, RootWebposts) |
id |
ID of the item. |
string |
isShared |
Indicates whether folder has been shared with someone. |
boolean |
lastUpdateTime |
Last time this item changed |
string |
name |
The name of this item. |
string |
parentId |
The ID of the parent folder. |
string |
path |
Path to this item. |
string |
size |
Size of this item in bytes. |
integer (int64) |
type |
Content Type. |
enum (Folder, File) |
6.16. FolderContentSqlRequest
Name | Description | Schema |
---|---|---|
id |
Parent Folder ID |
string |
name |
Name mask to search by |
string |
page |
Page number to display |
integer (int32) |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
sortDirection |
Sort direction; Default is ascending. |
string |
sortField |
Field to sort by; (type, time, size or name); Sorting order starts with type and ends with name |
string |
6.17. FolderDetailsModel
Represents a folder and all available attributes
Name | Description | Schema |
---|---|---|
allowFileOverwrite |
Indicates whether files of the same name will silently overwrite existing files of the same name. |
boolean |
cleanTimeDays |
Describes after how many days old files in this folder will be cleaned up. |
integer (int32) |
cleanType |
Describes what kind of automated clean up is regularly performed on this folder. |
enum (Never, AfterCleanTime) |
deliveryRecepientNotificationType |
Describes what kind of delivery recipient notifications are used in this folder. |
enum (None, Immediately) |
description |
Description of this folder. |
string |
enforceUniqueFilenames |
Indicates whether files in this folder must have unique names. |
boolean |
fileMaskRule |
Indicates whether files uploaded to the folder that match the configured filemasks will be allowed or denied. |
enum (AllowExceptMatching, DenyExceptMatching) |
fileMasks |
Comma-delimited list of filemasks which all files uploaded to the folder will be matched against. |
string |
folderType |
Indicates what kind of folder this is. |
enum (Virtual, Normal, Archive, Webpost, GlobalMessaging, Mailbox, Root, RootArchives, RootWebposts) |
id |
The unique ID of this folder. |
string |
isShared |
Indicates whether this folder is shared with someone |
boolean |
lastContentChangeTime |
Last time the folder content changed |
string |
name |
The (short) name of this folder. |
string |
newFileNotificationTime |
Describes how often new file batch notifications are sent. |
integer (int32) |
newFileNotificationType |
Describes what kind of new file notifications are used in this folder. |
enum (None, Batch, Immediately) |
newTime |
The maximum amount of time, in days, that files in this folder are considered "new". |
integer (int32) |
orgId |
ID of the organization this folder exists in. |
integer (int32) |
owner |
The username of this folder’s owner. (Home folders only) |
string |
parentId |
The ID of this folder’s parent folder or "0" if it does not have a parent folder. |
string |
parentInheritRights |
Indicates whether the folder inherits its permissions from its parent folder. |
integer (int32) |
path |
The (long) name of this folder. |
string |
permission |
A MOVEit Permission describing the currently authorized user’s permissions on this folder. |
|
quota |
The folder quota, if any, in bytes. |
integer (int64) |
responseText |
For webpost folders, text to display after a post to this folder. |
string |
responseTime |
For webpost folders, the time after which redirection occurs. |
integer (int32) |
responseType |
For webpost folders, the webpost completion and redirection setting. |
enum (RedirectImmediately, RedirectAfter) |
subfolderCleanTime |
Describes after how many days empty subfolders in this folder will be cleaned up. |
integer (int32) |
systemType |
Indicates if this folder is under the control of users or the MOVEit Transfer system. |
enum (User, System) |
uploadNotificationConfirmationTime |
Describes how often batch notifications are sent. |
integer (int32) |
uploadNotificationConfirmationType |
Describes what kind of upload confirmation notifications are used in this folder. |
enum (None, Batch, Immediately) |
6.18. FolderPatchModel
Folder Update parameters
Name | Description | Schema |
---|---|---|
name |
New file name to set |
string |
6.19. FolderRequest
Name | Description | Schema |
---|---|---|
id |
Folder ID |
string |
6.20. GetFileListRequest
Name | Description | Schema |
---|---|---|
page |
Page number to display |
integer (int32) |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
sortDirection |
Sort direction; Default is ascending. |
string |
sortField |
Name of field to sort the results by. (name, size, datetime, uploadstamp or path); Default is Path. |
string |
6.21. GetFolderListRequest
Get all folders query
Name | Description | Schema |
---|---|---|
name |
File name mask for filtering list of Folders |
string |
page |
Page number to display |
integer (int32) |
path |
File path mask for filtering list of Folders |
string |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
sortDirection |
Sort direction; Default is ascending. |
string |
sortField |
Name of field to sort the results by. (Name, Type or Path); Default is Path. |
string |
6.22. GetGroupMemberListRequest
Name | Description | Schema |
---|---|---|
id |
Group ID |
string |
page |
Page number to display |
integer (int32) |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
6.23. GetGroupsListRequest
Represents requred information in order to create a request to retreive list of groups.
Name | Description | Schema |
---|---|---|
page |
Page number to display |
integer (int32) |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
6.24. GetSubfilesListRequest
Name | Description | Schema |
---|---|---|
id |
Parent Folder ID |
string |
name |
File Name mask to search by |
string |
page |
Page number to display |
integer (int32) |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
sortDirection |
Sort direction; Default is ascending. |
string |
sortField |
Field to sort by; Default is name. |
string |
6.25. GetSubfoldersListRequest
Name | Description | Schema |
---|---|---|
id |
Parent Folder ID |
string |
name |
Folder Name mask to search by |
string |
page |
Page number to display |
integer (int32) |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
sortDirection |
Sort direction; Default is ascending. |
string |
sortField |
Field to sort by; Default is name. |
string |
6.26. GetTransferStatusListRequest
Represents requred information in order to create a request to retreive list of XferStatus. If several filtering criteria are provided they are matched using AND logic, e.g. File Name is "abc" and userRealName is "John"
Name | Description | Schema |
---|---|---|
fileName |
Filter expression for File name |
string |
folderName |
Filter expression for Folder name |
string |
page |
Page number to display |
integer (int32) |
perPage |
Items per page in result collection |
integer (int32) |
recentlyCompletedPeriod |
Time period in seconds to return recently completed data. Default: 0 |
integer (int32) |
search |
Filter expression for several fields. Fields are matched with OR logic. AND logic is used if you provide expression for each particular query. Fields being matched: userLoginName, userRealName, userIp, folderName, fileName |
string |
sortDirection |
Sort direction; Default is ascending. |
string |
sortField |
Name of field to sort the results by. |
string |
statusDistributionPeriod |
Time period in seconds to calculate status distribution for. Default: RecentlyCompletedPeriod |
integer (int32) |
transferStatus |
Filter for status. Uses OR logic |
< enum (Failed, Stalled, Active, Completed) > array |
userFullName |
Filter expression for User Display name |
string |
userIp |
Filter expression for User IP |
string |
userLoginName |
Filter expression for Login name |
string |
userRealName |
Filter expression for User Display name |
string |
6.27. GroupModel
Represents group’s requested information.
Name | Description | Schema |
---|---|---|
description |
Additional information, related to the group. |
string |
id |
Unique ID for this group. |
integer (int32) |
membersCount |
Amount of users, who are members of this particular group. |
integer (int32) |
name |
Name of the group. |
string |
6.28. IFolderPermission
Represents folder permission object
Name | Description | Schema |
---|---|---|
canAddSubfolders |
User can add subfolders to the folder. |
boolean |
canChangeSettings |
User can change the settings of the folder. |
boolean |
canDelete |
User can delete the folder. |
boolean |
canDeleteFiles |
User can delete files in the folder. |
boolean |
canListFiles |
User can list the files in the folder. |
boolean |
canListSubfolders |
User can list the subfolders of a folder. |
boolean |
canReadFiles |
User can download the files in the folder. |
boolean |
canShare |
User can share files in the folder |
boolean |
canWriteFiles |
User can upload files to the folder. |
boolean |
6.29. OrgInfoDto
Organization information class.
Name | Description | Schema |
---|---|---|
allowPassChangeRequests |
Organization allow password change requirement setting |
boolean |
baseURL |
Organization base URL |
string |
contactLink |
Organization contact link in default language |
string |
contactLinkName |
Organization contact link name in default language |
string |
formattingLocale |
A Locale which is used for formatting dates and times in this organization. |
string |
id |
Organization ID |
string |
informationLink |
Organization information link in default language |
string |
informationLinkName |
Organization information link name in default language |
string |
language |
Organization language |
string |
mobileURL |
Organization mobile URL |
string |
name |
Organization name |
string |
securityBanner |
Organization security banner in default language |
string |
securityNotice |
Organization security notice in default language |
string |
securityNoticeRequired |
Organization security notice requirement setting |
enum (NotRequired, WhenNew, Always) |
securityNoticeStamp |
Organization security notice change stamp |
string |
techEmail |
Organization tech support email in default language |
string |
techInfo |
Organization tech support notice in default language |
string |
techName |
Organization tech support name in default language |
string |
techPhone |
Organization tech support phone in default language |
string |
6.30. PagedModel_FileDetailsModel_
Represents paged response object with proper navigations
Name | Description | Schema |
---|---|---|
items |
Items on the given page |
< FileDetailsModel > array |
paging |
Paging data for given response |
|
sorting |
Sorting data for given response |
< SortFieldDto > array |
6.31. PagedModel_FolderAclEntryModel_
Represents paged response object with proper navigations
Name | Description | Schema |
---|---|---|
items |
Items on the given page |
< FolderAclEntryModel > array |
paging |
Paging data for given response |
|
sorting |
Sorting data for given response |
< SortFieldDto > array |
6.32. PagedModel_FolderContentItemModel_
Represents paged response object with proper navigations
Name | Description | Schema |
---|---|---|
items |
Items on the given page |
< FolderContentItemModel > array |
paging |
Paging data for given response |
|
sorting |
Sorting data for given response |
< SortFieldDto > array |
6.33. PagedModel_GroupModel_
Represents paged response object with proper navigations
Name | Description | Schema |
---|---|---|
items |
Items on the given page |
< GroupModel > array |
paging |
Paging data for given response |
|
sorting |
Sorting data for given response |
< SortFieldDto > array |
6.34. PagedModel_SimpleFileModel_
Represents paged response object with proper navigations
Name | Description | Schema |
---|---|---|
items |
Items on the given page |
< SimpleFileModel > array |
paging |
Paging data for given response |
|
sorting |
Sorting data for given response |
< SortFieldDto > array |
6.35. PagedModel_SimpleFolderModel_
Represents paged response object with proper navigations
Name | Description | Schema |
---|---|---|
items |
Items on the given page |
< SimpleFolderModel > array |
paging |
Paging data for given response |
|
sorting |
Sorting data for given response |
< SortFieldDto > array |
6.36. PagedModel_SimpleUserModel_
Represents paged response object with proper navigations
Name | Description | Schema |
---|---|---|
items |
Items on the given page |
< SimpleUserModel > array |
paging |
Paging data for given response |
|
sorting |
Sorting data for given response |
< SortFieldDto > array |
6.37. PagedModel_UserGroupModel_
Represents paged response object with proper navigations
Name | Description | Schema |
---|---|---|
items |
Items on the given page |
< UserGroupModel > array |
paging |
Paging data for given response |
|
sorting |
Sorting data for given response |
< SortFieldDto > array |
6.38. PagingInfoModel
Information about paging for collection response
Name | Description | Schema |
---|---|---|
page |
Current page in collection |
integer (int32) |
perPage |
Items per page |
integer (int32) |
totalItems |
Total items in collection |
integer (int64) |
totalPages |
Total number of pages in collection |
integer (int32) |
6.39. RequestTokenError
Authentication failed error
Name | Description | Schema |
---|---|---|
error |
Error title |
string |
error_description |
Error details |
string |
6.40. RevokeTokenResponse
Response for Revoke token operation
Name | Description | Schema |
---|---|---|
message |
Message |
string |
6.41. SimpleFileModel
Container object used to provide information about a specific file on a MOVEit Transfer server.
Name | Description | Schema |
---|---|---|
id |
The unique ID of this file. Also known as the tracking number. |
string |
name |
The name of this file. |
string |
path |
The full path of this file. |
string |
size |
Size in bytes of this file. |
integer (int64) |
uploadStamp |
The date and time this file was uploaded. |
string |
6.42. SimpleFolderModel
Represents basic folder data required for all scenarios
Name | Description | Schema |
---|---|---|
folderType |
Indicates what kind of folder this is. |
enum (Virtual, Normal, Archive, Webpost, GlobalMessaging, Mailbox, Root, RootArchives, RootWebposts) |
id |
The unique ID of this folder. |
string |
isShared |
Indicates whether this folder is shared with someone |
boolean |
lastContentChangeTime |
Last time the folder content changed |
string |
name |
The (short) name of this folder. |
string |
parentId |
The ID of this folder’s parent folder or "0" if it does not have a parent folder. |
string |
path |
The (long) name of this folder. |
string |
6.43. SimpleUserModel
Simple user model for user lists.
Name | Description | Schema |
---|---|---|
email |
User email address. |
string |
fullName |
User full name. |
string |
id |
User id. |
string |
lastLoginStamp |
Time of last user login. |
string |
orgID |
Id of organization user is a member of. |
integer (int32) |
permission |
User permission. |
enum (TemporaryUser, User, FileAdmin, Admin, SysAdmin) |
realname |
User full name. |
string |
status |
User security status. |
enum (Active, Suspended, Template) |
username |
User login name. |
string |
6.44. SortFieldDto
Name | Schema |
---|---|
sortDirection |
enum (asc, desc) |
sortField |
string |
6.45. TokenAcquiredModel
Represents successful token request response
Name | Description | Schema |
---|---|---|
access_token |
API Token |
string |
expires_in |
Number of seconds till token expiration |
integer (int32) |
refresh_token |
Refresh token |
string |
token_type |
Type of the token |
string |
6.46. TransferStatusDistribution
Distribution of available statuses in collection
Name | Description | Schema |
---|---|---|
active |
Number of records in Active state |
integer (int32) |
completed |
Number of records in Succeeded state |
integer (int32) |
failed |
Number of records in Failed state |
integer (int32) |
lingeringTransfers |
Number of transfers that have recently been completed but still are displayed as in progress |
integer (int32) |
stalled |
Number of records in Stalled state |
integer (int32) |
6.47. TransferStatusModel
Represents active transfer information
Name | Description | Schema |
---|---|---|
agentBrand |
User agent brand |
string |
agentVersion |
User agent version |
string |
currentBytes |
Bytes already transferred |
integer (int64) |
direction |
Transfer direction |
enum (Upload, Download) |
errorCode |
Information about the transfer status, such as an error code |
integer (int32) |
fileId |
ID of the file |
string |
fileName |
File Name |
string |
folderId |
Parent Folder ID |
string |
folderName |
Parent Folder Name |
string |
folderPath |
Parent Folder Path |
string |
folderType |
Parent Folder Type |
enum (Virtual, Normal, Archive, Webpost, GlobalMessaging, Mailbox, Root, RootArchives, RootWebposts) |
integrityVerified |
Integrity Verified |
boolean |
moduleName |
Module which performs transfer |
enum (Other, FTP, SSH, ISAPI, REST) |
nodeNum |
Web Farm node number |
integer (int32) |
orgId |
ID of organization this file belongs to |
integer (int32) |
statusMessage |
Information about the transfer status, such as an error message |
string |
timeEnded |
Time when transfer ended. Null if transfer is in progress |
string |
timeStarted |
Time when transfer started |
string |
totalBytes |
Total file size, if known |
integer (int64) |
transferRate |
Rate of the transfer |
integer (int64) |
transferStatus |
Transfer status |
enum (Failed, Stalled, Active, Completed) |
userFullName |
User Real Name |
string |
userId |
ID of the user |
string |
userIp |
IP Address of user |
string |
userLoginName |
User Login Name |
string |
userRealName |
User Real Name |
string |
6.48. TransferStatusPagedModel
Name | Description | Schema |
---|---|---|
items |
Items on the given page |
< TransferStatusModel > array |
paging |
Paging data for given response |
|
sorting |
Sorting data for given response |
< SortFieldDto > array |
statusDistribution |
Status distribution |
6.49. UnprocessableEntityErrorModel
Represents an error returned by API in case of HTTP 422 error
Name | Description | Schema |
---|---|---|
detail |
An explanation specific to this occurrence of the error |
string |
errorCode |
An error number, defined by the application |
integer (int32) |
errors |
Detailed information about errors in provided parameters |
< FieldErrorModel > array |
title |
Short summary of the error type |
string |
6.50. UserDetailsModel
Detailed user model.
Name | Description | Schema |
---|---|---|
authMethod |
User authentication method; only takes effect if the organization is set to "External then MOVEit" authentication. |
enum (MOVEitOnly, ExternalOnly, Both) |
defaultFolderID |
ID of user default folder; 0 indicates the home folder is the default folder. |
integer (int64) |
displaySettings |
||
email |
User email address. |
string |
emailFormat |
Format of email notifications user receives. |
enum (HTML, Text) |
expirationPolicyID |
ID of the expiration policy configured for this user, or 0 for no policy. |
integer (int32) |
folderQuota |
User quota value, or 0 for no quota. |
integer (int64) |
forceChangePassword |
If set to 1, user must change their password on their next login. |
boolean |
fullName |
User full name. |
string |
homeFolderID |
ID of user home folder, or 0 for no home folder. |
integer (int64) |
id |
User id. |
string |
language |
User language code. |
string |
lastLoginStamp |
Time of last user login. |
string |
notes |
User description. |
string |
orgID |
Id of organization user is a member of. |
integer (int32) |
passwordChangeStamp |
Time of last user password change. |
string |
permission |
User permission. |
enum (TemporaryUser, User, FileAdmin, Admin, SysAdmin) |
realname |
User full name. |
string |
receivesNotification |
User email notifications setting. |
enum (ReceivesNoNotifications, ReceivesNotifications, AdminReceivesNotifications) |
status |
User security status. |
enum (Active, Suspended, Template) |
statusNote |
User security status description. |
string |
username |
User login name. |
string |
6.51. UserGroupModel
Represents a group that a specific user is a member of, including the relationship that user has with the group.
Name | Description | Schema |
---|---|---|
description |
Additional information, related to the group. |
string |
id |
Unique ID for this group. |
integer (int32) |
name |
Name of the group. |
string |
relationship |
User Group relationship |
enum (Member, GroupAdmin) |
6.52. UserGroupsRequest
User list query.
Name | Description | Schema |
---|---|---|
id |
User Id. |
string |
page |
Page number to display |
integer (int32) |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
6.53. UserListRequest
User list query.
Name | Description | Schema |
---|---|---|
email |
User email |
string |
fullName |
User real name |
string |
page |
Page number to display |
integer (int32) |
perPage |
Items per page in result collection; Default is 25 |
integer (int32) |
permission |
User permission filter. |
enum (AllUsers, EndUsers, Administrators, FileAdmins, GroupAdmins, TemporaryUsers, SysAdmins) |
realname |
User real name |
string |
sortDirection |
Sort direction; Default is ascending. |
string |
sortField |
Name of field to sort the results by. (username, realname, lastLoginStamp or email); Default is username. |
string |
status |
User status filter. |
enum (AllUsers, ActiveUsers, InactiveUsers, NeverSignedOnUsers, TemplateUsers) |
username |
User name |
string |
6.54. UserPatchModel
Contains the user properties that should change
Name | Description | Schema |
---|---|---|
authMethod |
Auth method type |
enum (MOVEitOnly, ExternalOnly, Both) |
defaultFolderId |
Default folder Id |
integer (int64) |
email |
Email address |
string |
emailFormat |
Email format type |
enum (HTML, Text) |
folderQuota |
User quota |
integer (int64) |
forceChangePassword |
Force password change |
boolean |
fullName |
Real name of user |
string |
language |
Language |
string |
notes |
Notes |
string |
password |
User password |
string |
permission |
User permission type |
enum (TemporaryUser, User, FileAdmin, Admin, SysAdmin) |
realName |
Real name of user |
string |
receivesNotification |
Notification type |
enum (ReceivesNoNotifications, ReceivesNotifications, AdminReceivesNotifications) |
status |
User status type |
enum (Active, Suspended, Template) |
statusNote |
User status note |
string |
6.55. UserRequest
Name | Description | Schema |
---|---|---|
id |
User ID |
string |